基础
定义: 定义一个操作中算法的框架,而将一些步骤延迟到子类中,使得子类可以不改变算法的结构即可重定义该算法中的某些特定步骤。
类型: 行为类模式
关键点: 定义一个算法结构,而将一些步骤延迟到子类实现。
类图
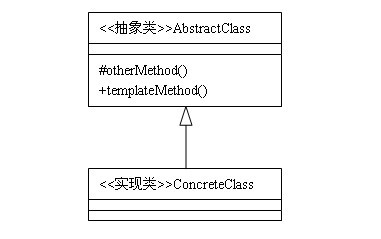
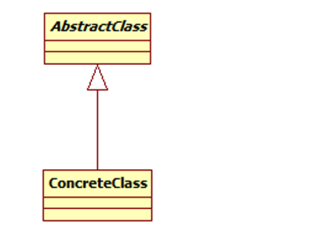
Java代码
abstract class AbstractSort {
protected abstract void sort(int[] array);
public void showSortResult(int[] array) {
this.sort(array);
System.out.print("排序结果:");
for (int i = 0; i < array.length; i++) {
System.out.printf("%3s", array[i]);
}
}
}
class ConcreteSort extends AbstractSort {
@Override
protected void sort(int[] array) {
for(int i = 0; i < array.length - 1; i++){
selectSort(array, i);
}
}
private void selectSort(int[] array, int index) {
// 最小值变量
int minValue = 32767;
// 最小值索引变量
int indexMin = 0;
int temp; // 暂存变量
for (int i = index; i < array.length; i++) {
// 找到最小值
if (array[i] < minValue) {
// 储存最小值
minValue = array[i];
indexMin = i;
}
}
// 交换两数值
temp = array[index];
array[index] = array[indexMin];
array[indexMin] = temp;
}
}
class Client {
public static void main(String[] args) {
// 预设数据数组
int[] a = {10, 32, 1, 9, 5, 7, 12, 0, 4, 3};
AbstractSort s = new ConcreteSort();
s.showSortResult(a);
}
}
PHP代码
abstract class AbstractSort
{
abstract protected function sort(&$array);
public function showSortResult($array)
{
$this->sort($array);
echo "排序结果:";
foreach ($array as $value) {
echo sprintf("%3s", $value);
}
}
}
class ConcreteSort extends AbstractSort
{
protected function sort(&$array)
{
sort($array);
}
}
class TemplateMethodTest extends TestCase
{
public function test()
{
$array = [10, 32, 1, 9, 5, 7, 12, 0, 4, 3];
$s = new ConcreteSort();
$s->showSortResult($array);
}
}
执行结果
排序结果: 0 1 3 4 5 7 9 10 12 32